直接利用剪切板处理B站网页视频链接
程序源码
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <windows.h>
//将内容写入剪切板
int write_clipboard(char *strText);
//从剪切板中读取内容
int get_clipboard(char *str);
int main(void){
//定义从剪切板传入的字符串
char import[195] = {0};
//从剪切板中截取视频识别相关代码
char middle[42] = {0};
//定义一个总长为三段相加的空字符串
char full[302] = {0};
//字符串的头部,即处理之后的链接的前部分,写死在程序中
char *start = (char *)"<iframe src=\"//player.bilibili.com/player.html?aid=";
//字符串的结尾,即处理之后的链接的后部分,写死在程序中
char *end = (char *)"&page=1&high_quality=1&danmaku=0\" allowfullscreen=\"allowfullscreen\" width=\"100%\" height=\"500\" scrolling=\"no\" frameborder=\"0\" sandbox=\"allow-top-navigation allow-same-origin allow-forms allow-scripts\"></iframe>";
//从剪切板读取字符传入import
get_clipboard(import);
//字符串的中间部分,作测试用
//char *middle = (char *)"375588815&bvid=BV1so4y1m7U5&cid=339262048";
//获取字符串的中间部分,放入middle中
for(int i = 0; i<41; i++){
middle[i] = import[51+i];
}
//将字符串的各部分连接起来放到full中
strcat(full,start);
strcat(full,middle);
strcat(full,end);
//将字符串输出测试
printf("%s",full);
//将处理完成的字符串写入到剪切板
write_clipboard(full);
//系统暂停,浏览输出结果
system("pause");
return 0;
}
int write_clipboard(char *strText)
{
// 打开剪贴板
if (!OpenClipboard(NULL)|| !EmptyClipboard())
{
printf("打开或清空剪切板出错!\n");
return -1;
}
HGLOBAL hMen;
// 分配全局内存
hMen = GlobalAlloc(GMEM_MOVEABLE, ((strlen(strText)+1)*sizeof(TCHAR)));
if (!hMen)
{
printf("分配全局内存出错!\n");
// 关闭剪切板
CloseClipboard();
return -1;
}
// 把数据拷贝考全局内存中
// 锁住内存区
LPSTR lpStr = (LPSTR)GlobalLock(hMen);
// 内存复制
memcpy(lpStr, strText, ((strlen(strText))*sizeof(TCHAR)));
// 字符结束符
lpStr[strlen(strText)] = (TCHAR)0;
// 释放锁
GlobalUnlock(hMen);
// 把内存中的数据放到剪切板上
SetClipboardData(CF_TEXT, hMen);
CloseClipboard();
return 0;
}
int get_clipboard(char *str){
//局部变量
char *pbuf = NULL;
//剪切板句柄
HANDLE hclip;
//打开剪切板,获取里面的数据
if(OpenClipboard(NULL) == 0){
printf("打开剪切板失败!\n");
return -1;
}
//判断剪切板当中的数据是不是文本类型的
if(!IsClipboardFormatAvailable(CF_TEXT)){
printf("剪切板当中的数据类型不匹配!\n");
//关闭剪切板,不然其他程序无法正常使用剪切板
CloseClipboard();
return -1;
}
//获取剪切板里面的数据
hclip = GetClipboardData(CF_TEXT);
//加锁,返回一个VOID类型的指针
pbuf = (char *)GlobalLock(hclip);
//解锁
GlobalUnlock(hclip);
//将剪切板里面所有字符传入给参数
while(1){
//如果到了字符串末尾就退出循环
if(*pbuf == 0){
break;
}
//定义一个用于循环的局部变量
int i = 0;
do{
//将读取到的字母或数字字符赋给word
str[i] = *pbuf;
//将指针指向下一个字符处
++pbuf;
++i;
}while(*pbuf);
//添加字符串结束
str[i] = '\0';
}
//关闭剪切板,不然其他程序无法正常0使用剪切板
CloseClipboard();
return 0;
}
程序运行结果示例
不打印输出内容,黑框直接一闪而过版本
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <windows.h>
//将内容写入剪切板
int write_clipboard(char *strText);
//从剪切板中读取内容
int get_clipboard(char *str);
int main(void){
//定义从剪切板传入的字符串
char import[195] = {0};
//从剪切板中截取视频识别相关代码
char middle[42] = {0};
//定义一个总长为三段相加的空字符串
char full[302] = {0};
//字符串的头部,即处理之后的链接的前部分,写死在程序中
char *start = (char *)"<iframe src=\"//player.bilibili.com/player.html?aid=";
//字符串的结尾,即处理之后的链接的后部分,写死在程序中
char *end = (char *)"&page=1&high_quality=1&danmaku=0\" allowfullscreen=\"allowfullscreen\" width=\"100%\" height=\"500\" scrolling=\"no\" frameborder=\"0\" sandbox=\"allow-top-navigation allow-same-origin allow-forms allow-scripts\"></iframe>";
//从剪切板读取字符传入import
get_clipboard(import);
//字符串的中间部分,作测试用
//char *middle = (char *)"375588815&bvid=BV1so4y1m7U5&cid=339262048";
//获取字符串的中间部分,放入middle中
for(int i = 0; i<41; i++){
middle[i] = import[51+i];
}
//将字符串的各部分连接起来放到full中
strcat(full,start);
strcat(full,middle);
strcat(full,end);
//将字符串输出测试
//printf("%s",full);
//将处理完成的字符串写入到剪切板
write_clipboard(full);
//系统暂停,浏览输出结果
//system("pause");
return 0;
}
int write_clipboard(char *strText)
{
// 打开剪贴板
if (!OpenClipboard(NULL)|| !EmptyClipboard())
{
printf("打开或清空剪切板出错!\n");
return -1;
}
HGLOBAL hMen;
// 分配全局内存
hMen = GlobalAlloc(GMEM_MOVEABLE, ((strlen(strText)+1)*sizeof(TCHAR)));
if (!hMen)
{
printf("分配全局内存出错!\n");
// 关闭剪切板
CloseClipboard();
return -1;
}
// 把数据拷贝考全局内存中
// 锁住内存区
LPSTR lpStr = (LPSTR)GlobalLock(hMen);
// 内存复制
memcpy(lpStr, strText, ((strlen(strText))*sizeof(TCHAR)));
// 字符结束符
lpStr[strlen(strText)] = (TCHAR)0;
// 释放锁
GlobalUnlock(hMen);
// 把内存中的数据放到剪切板上
SetClipboardData(CF_TEXT, hMen);
CloseClipboard();
return 0;
}
int get_clipboard(char *str){
//局部变量
char *pbuf = NULL;
//剪切板句柄
HANDLE hclip;
//打开剪切板,获取里面的数据
if(OpenClipboard(NULL) == 0){
printf("打开剪切板失败!\n");
return -1;
}
//判断剪切板当中的数据是不是文本类型的
if(!IsClipboardFormatAvailable(CF_TEXT)){
printf("剪切板当中的数据类型不匹配!\n");
//关闭剪切板,不然其他程序无法正常使用剪切板
CloseClipboard();
return -1;
}
//获取剪切板里面的数据
hclip = GetClipboardData(CF_TEXT);
//加锁,返回一个VOID类型的指针
pbuf = (char *)GlobalLock(hclip);
//解锁
GlobalUnlock(hclip);
//将剪切板里面所有字符传入给参数
while(1){
//如果到了字符串末尾就退出循环
if(*pbuf == 0){
break;
}
//定义一个用于循环的局部变量
int i = 0;
do{
//将读取到的字母或数字字符赋给word
str[i] = *pbuf;
//将指针指向下一个字符处
++pbuf;
++i;
}while(*pbuf);
//添加字符串结束
str[i] = '\0';
}
//关闭剪切板,不然其他程序无法正常0使用剪切板
CloseClipboard();
return 0;
}
THE END
0
二维码
海报
直接利用剪切板处理B站网页视频链接
程序源码
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <windows.h>
//将内容写入剪切板
int write……
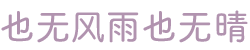
共有 0 条评论